Response:
Add a sketch with a tool, located in the icon on the top left corner. Afterwards click the button to check the sketches.
Click on "setSketchUpdate" to receive a message every time, a sketch is being updated.
Have a look at the sketching example on this page, to see how to use the sketching tool.
Have a look at the Code example on this page, to see how to change the zoom animations.
Read more detailed information in the referenced API documentation:
getAllCreatedObjectsFromMap API reference zoomToAllGraphicObjects API reference setSketchUpdate API reference getMapExtentFromAllCreatedObjects API referenceTo use the function as seen in the example, you have to open the toolbar.

and choose the selection tool.
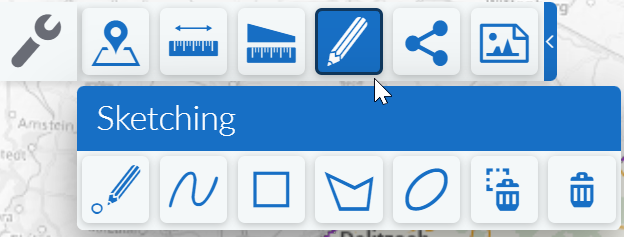
Now you can choose between different sketching tools.
For Polygons: With a click you can start to set set Points. Use a double click to end the sequence of points.
To use the function as seen in the example, use following code:
function postEvent(functionName, detail) {
const integrationElement = document.getElementById('mapIntegrationElement').contentWindow
if(integrationElement){
integrationElement.postMessage({functionName: functionName, detail: detail}, "*")
}
}
[...]
// zoom to the objects
postEvent("getAllCreatedObjectsFromMap",{})
//zoom to the objects with custom settings
postEvent("zoomToAllGraphicObjects",
{
animations: {
animate: true,
duration: 6000,
easing: "ease"
},
scale: 50000
}
)
[...]
// Listen to the events
window.addEventListener(
"message",
(evt) => {
if(evt.data.functionName === "getAllCreatedObjectsFromMap"){
console.log(evt)
} else if (evt.data.functionName === "setSketchUpdate") {
console.log(evt)
}
},
false
);
To delete sketches, you can use following example:
function postEvent(functionName, detail) {
const integrationElement = document.getElementById('mapIntegrationElement').contentWindow
if(integrationElement){
integrationElement.postMessage({functionName: functionName, detail: detail}, "*")
}
}
[...]
// delete multiple graphics
const parameterArray = [1,2,3]
postEvent("clearGraphics",{id: parameterArray, layerId: "sketch-graphics"})
[...]
// delete single graphic
const parameter = 4
postEvent("clearGraphics", {id: parameter, layerId: "sketch-graphics"})
[...]
// delete all graphics
postEvent("clearAllGraphics",{layerId: "sketch-graphics"})